Graph Editor | Connect with Rhino Grasshopper
Introduction
In addition to using Twikit's built-in modifiers, users have the option to link with their native Rhino Grasshopper scripts (https://www.rhino3d.com/). Grasshopper, a widely-used tool for parametrization, is a visual programming language that operations within the Rhinoceros CAD application.
Grasshopper scripts can be uploaded to the Graph Editor as GHX files under the Resources section. Detailed information on uploading and managing resources in the graph editor is available inGraph editor | Resources.
To integrate the script into the graph, utilize the 'Twikit Connect' modifier located in the 3D modifier group.
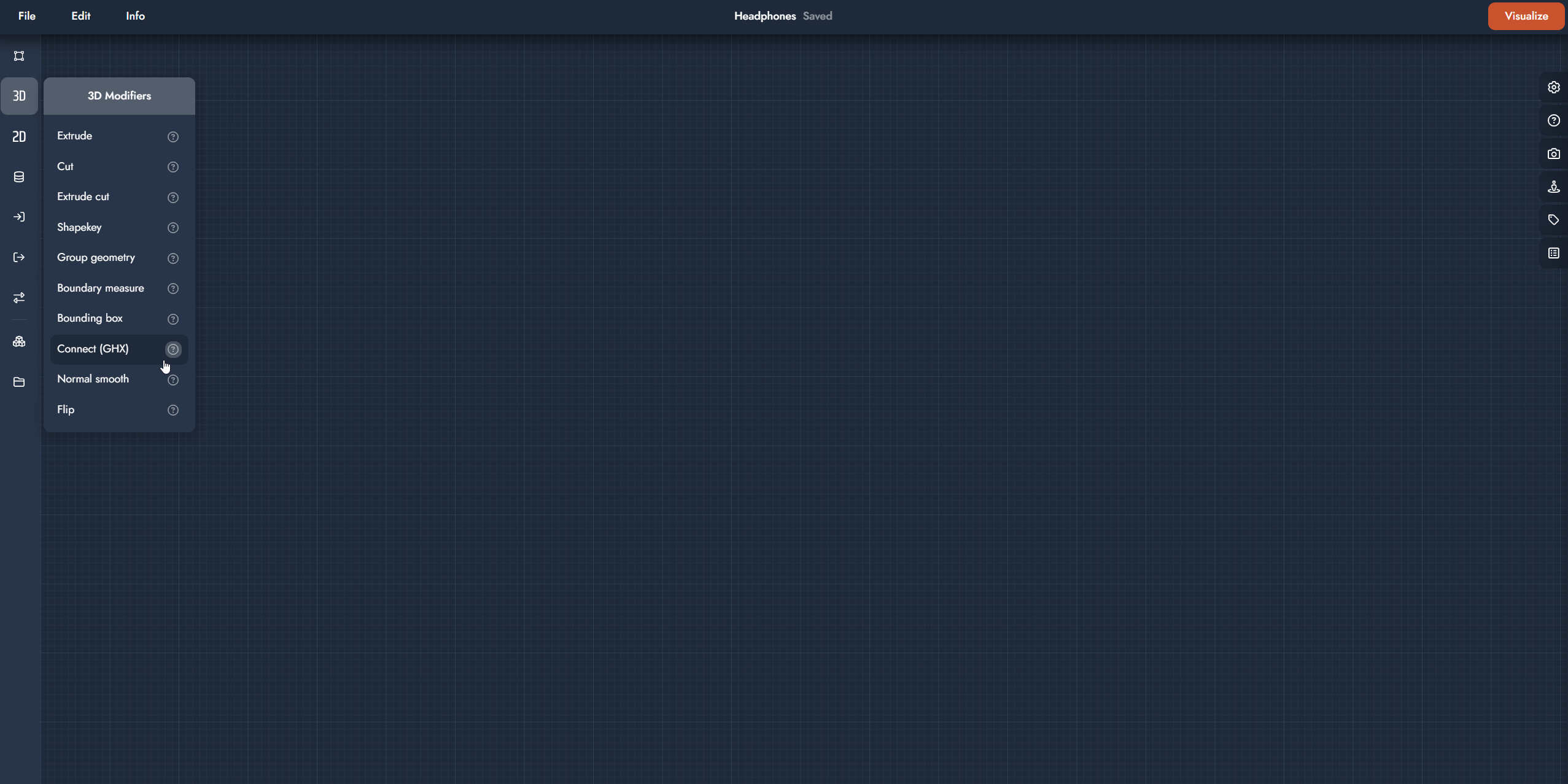
Accessing the Connect modifier
The use of in- and outputs
Integrating Grasshopper with TwikBot 5 requires specific input and output configurations to facilitate communication between the two platforms. Twikit Connect is compatible with a broad range of inputs and outputs, provided they are properly set up.
It's important to note that Twikit Connect will override any pre-configured input parameters with data from TwikBot 5. For instance, while a slider connected to a number input parameter may be useful for testing in Grasshopper during the design process, its value becomes irrelevant in TwikBot 5. In TwikBot 5, the number input will be replaced by the value received from the platform, superseding the slider's setting.
Base configuration
To make a Grasshopper input or output accessible to Twikbot 5, place the parameter of the respective item in a dedicated group. Name this group 'RH_IN: [Unique name]' for inputs or 'RH_OUT: [Unique name]' for outputs, depending on its function."
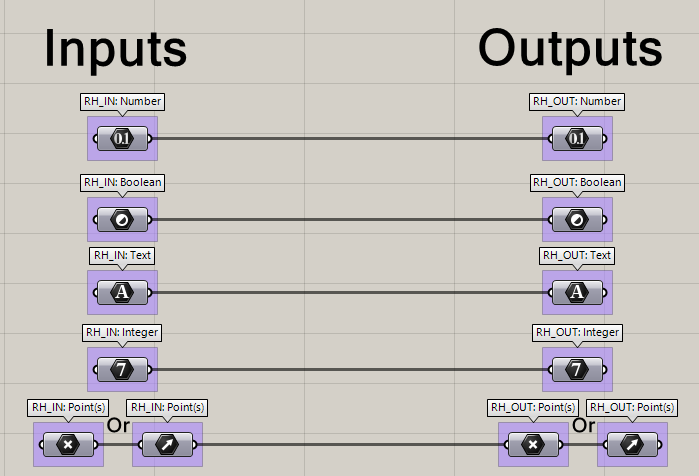
The recommended configuration for inputs and outputs in Grasshopper.
Mesh configuration
Meshes require a unique configuration, particularly for inputs. These are initially managed via a text parameter and then converted through a C# modifier. It's advised to utilize the standard mesh parameter during design and switch to a C# modifier before exporting the script for TwikBot 5 integration. For outputs, the primary distinction lies in the group naming convention, which should be 'RH_OUT:mesh: [Unique name]'.
A mesh output should always be one joined, manifold mesh.
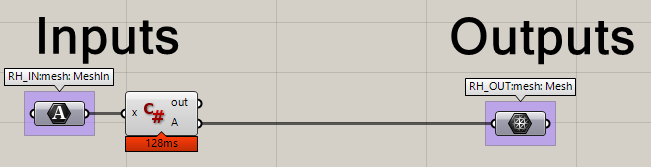
The commended configuration of mesh inputs and outputs in Grasshopper.
The required code to be included in the C# script is provided below:
using System;
using System.Collections;
using System.Collections.Generic;
using Rhino;
using Rhino.Geometry;
using Grasshopper;
using Grasshopper.Kernel;
using Grasshopper.Kernel.Data;
using Grasshopper.Kernel.Types;
using System.IO;
using System.Linq;
/// <summary>
/// This class will be instantiated on demand by the Script component.
/// </summary>
public class Script_Instance : GH_ScriptInstance
{
#region Utility functions
/// <summary>Print a String to the [Out] Parameter of the Script component.</summary>
/// <param name="text">String to print.</param>
private void Print(string text) { /* Implementation hidden. */ }
/// <summary>Print a formatted String to the [Out] Parameter of the Script component.</summary>
/// <param name="format">String format.</param>
/// <param name="args">Formatting parameters.</param>
private void Print(string format, params object[] args) { /* Implementation hidden. */ }
/// <summary>Print useful information about an object instance to the [Out] Parameter of the Script component. </summary>
/// <param name="obj">Object instance to parse.</param>
private void Reflect(object obj) { /* Implementation hidden. */ }
/// <summary>Print the signatures of all the overloads of a specific method to the [Out] Parameter of the Script component. </summary>
/// <param name="obj">Object instance to parse.</param>
private void Reflect(object obj, string method_name) { /* Implementation hidden. */ }
#endregion
#region Members
/// <summary>Gets the current Rhino document.</summary>
private readonly RhinoDoc RhinoDocument;
/// <summary>Gets the Grasshopper document that owns this script.</summary>
private readonly GH_Document GrasshopperDocument;
/// <summary>Gets the Grasshopper script component that owns this script.</summary>
private readonly IGH_Component Component;
/// <summary>
/// Gets the current iteration count. The first call to RunScript() is associated with Iteration==0.
/// Any subsequent call within the same solution will increment the Iteration count.
/// </summary>
private readonly int Iteration;
#endregion
/// <summary>
/// This procedure contains the user code. Input parameters are provided as regular arguments,
/// Output parameters as ref arguments. You don't have to assign output parameters,
/// they will have a default value.
/// </summary>
private void RunScript(string x, ref object A)
{
var decodedData = Convert.FromBase64String(x); //the obj file
var filename = Path.GetTempFileName();
filename = Path.ChangeExtension(filename, ".obj"); // the tmp obj file name on disk
File.WriteAllBytes(filename, decodedData); //write the file to disk
var doc = Rhino.RhinoDoc.CreateHeadless(null); // create a Rhino File
doc.Import(filename); // import the obj into this file
var objects = doc.Objects.GetObjectList(Rhino.DocObjects.ObjectType.Mesh); // get the imported meshes
Mesh geometry = objects.SingleOrDefault().Geometry as Mesh; // get the mesh
A = geometry; // return the mesh
}
// <Custom additional code>
// </Custom additional code>
}
Best practices
The best practices outlined below are known to be effective for working with Grasshopper scripts in TwikBot 5.
Clean file
A clean file minimizes unexpected issues and reduces debugging time. Key aspects of maintaining a clean file include:
Remove unused modifiers: Avoid keeping stray modifiers in your script as they can cause errors or warnings, potentially leading to incompatibility.
Internalize data: Ensure that all data is internalized. Failing to do so can result in data loss when the Grasshopper file is saved.
Organize inputs and outputs: Group all input parameters at the beginning of the script and outputs at the right end. This arrangement aids in script readability.
Script flow from left to right: Since most people naturally read from left to right, structuring your script in this manner can simplify debugging when necessary.
Data Dams
Data dams are invaluable tools in script development, acting as checkpoints that halt data flow through a modifier unless explicitly opened. This feature prevents the script from recomputing entirely each time a modification is made at the beginning of the script.
However, with Twikit Connect, it's essential for the script to recompute fully whenever any of its parameters change. Therefore, before importing the script into TwikBot 5, ensure that all data dams are set to 'Always Open'. This setting ensures that the script responds appropriately to parameter adjustments within TwikBot 5
The use of Grasshopper plugins
Grasshopper's strength is greatly enhanced by its wide range of available plugins, catering to diverse needs such as complex calculations or object re-meshing based on specific algorithms.
However, it's important to recognize plugin compatibility with Twikit Connect. Plugins that are unsupported cannot be integrated with Twikit Connect. The reasons vary and may include legal constraints among others.
Tracking Plugins in Grasshopper with MetaHopper
Maintaining an overview of all the plugins used in a Grasshopper script can be challenging. Fortunately, this task can be simplified using the MetaHopper plugin (https://www.food4rhino.com/en/app/metahopper). The 'Document Info' component of MetaHopper particularly its '3rd Party Assemblies (A)' parameter, provides a list of all third-party plugins present in the document, excluding the 'Document Info' component itself. This feature is instrumental in efficiently tracking and managing the plugins used in your Grasshopper script.
Don’t forget to delete the component for checking the plugins before importing the script in TwikBot 5.
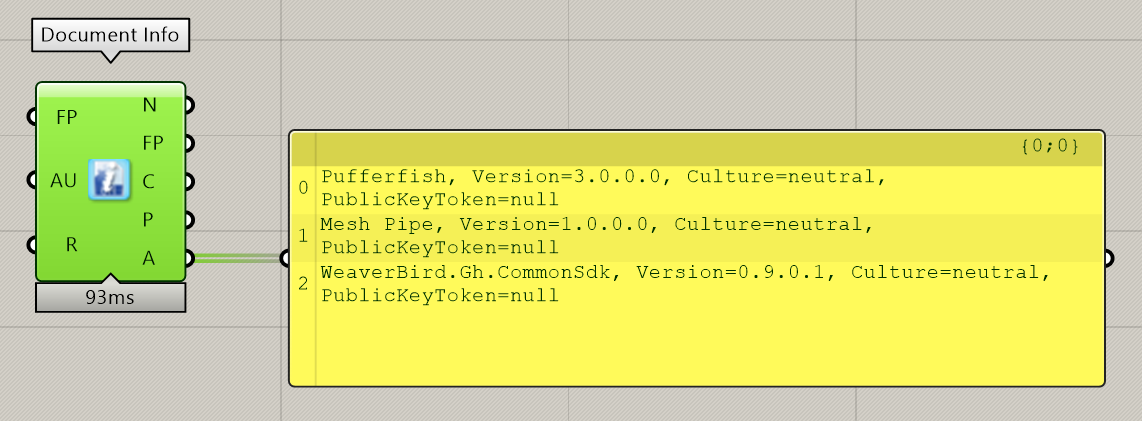
The Document Info component
Supported plugins
Name |
---|
Dendro |
Jellyfish |
Human |
LunchBox |
Pufferfish |
Shortest Walk |
Weaverbird |
FEA |
Intralattice |
Meshedit |
Meshtools |